Demystifying Distributed Systems
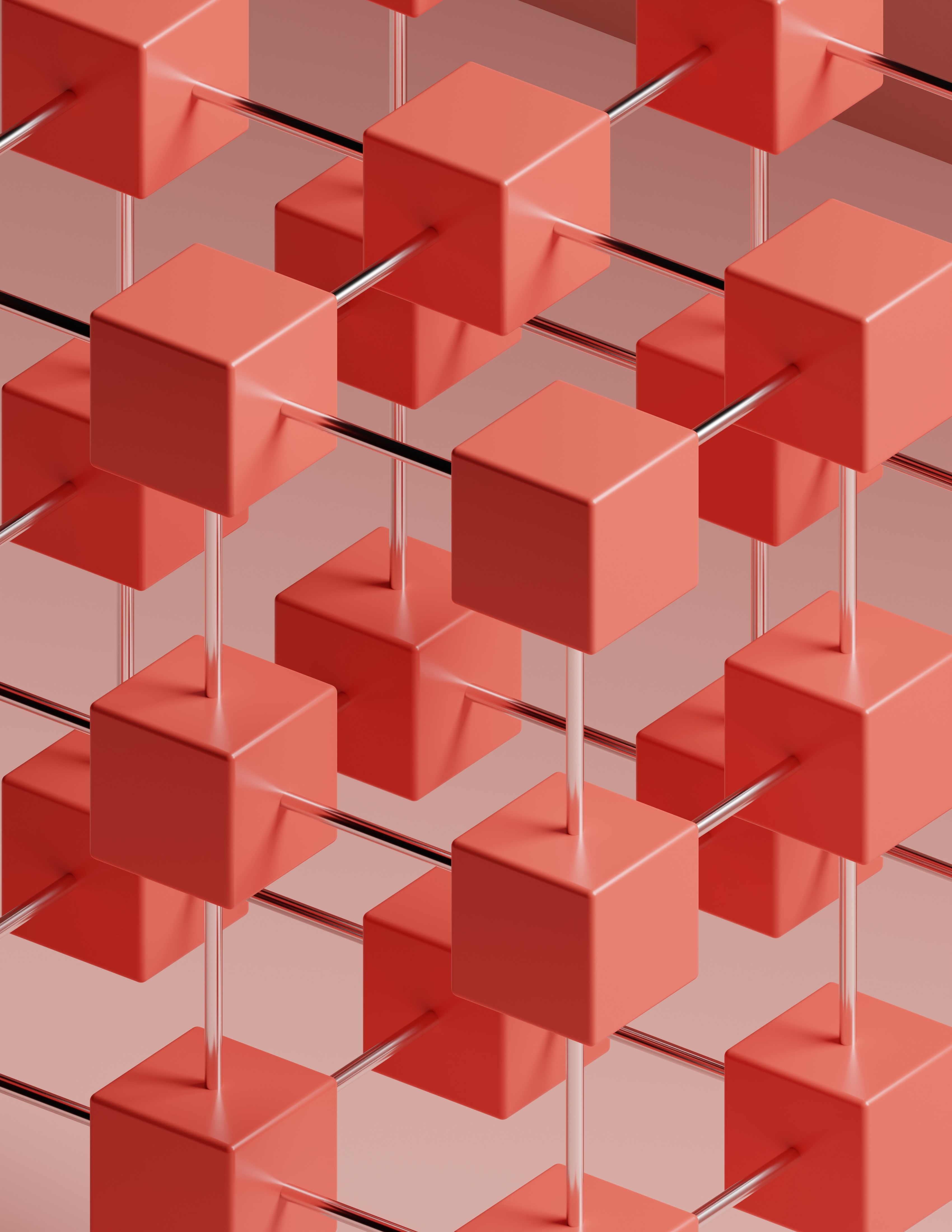
Distributed systems are becoming increasingly popular in today’s world where applications need to handle massive amounts of data and traffic. A distributed system is a collection of autonomous computers connected by a network that communicate and coordinate their actions to achieve a common goal. In this post, we’ll explore the concept and principles of distributed systems and provide examples in Node.js, a popular runtime environment for building scalable and efficient distributed systems.
Principles of Distributed Systems
The principles of distributed systems are essential for designing and implementing a system that is fault-tolerant, highly available, and scalable. Here are some of the most critical principles:
-
Decentralization: In a distributed system, there is no central authority or point of control. Instead, each computer in the system is independent and self-governing, and all nodes work together to achieve a common goal.
-
Redundancy: A distributed system should have multiple copies of data and services to ensure that if one node fails, there are still other nodes available to perform the same task.
-
Consistency: All nodes in the distributed system should have access to the same data and services, regardless of their location or time. Achieving consistency requires synchronization and coordination between nodes.
Example of a Distributed System in Node.js
Let’s consider an example of a distributed system in Node.js. Suppose you have an online store that receives millions of requests every day. You need a system that can handle this volume of traffic while ensuring that the application is fault-tolerant and highly available.
Here’s how you can build a distributed system in Node.js:
- Use a message queue: A message queue is a tool that helps you manage communication between different components of your distributed system. In our example, we can use RabbitMQ, a popular message queue, to handle incoming requests and distribute them across multiple nodes.
We’ll take RabbitMQ as a message queue example. RabbitMQ is a popular message queue that is widely used in distributed systems. Here’s an example of how to use RabbitMQ with Node.js to manage communication between different components of a system:
// Import the amqplib library
const amqp = require('amqplib');
// Connect to RabbitMQ server
amqp.connect('amqp://localhost')
.then(function(connection) {
// Create a channel
return connection.createChannel()
.then(function(channel) {
// Declare a queue
const queue = 'my-queue';
return channel.assertQueue(queue, { durable: true })
.then(function() {
// Send a message to the queue
const message = 'Hello, world!';
channel.sendToQueue(queue, Buffer.from(message), { persistent: true });
console.log("Sent '%s' to '%s'", message, queue);
});
})
.finally(function() { connection.close(); });
})
.catch(function(error) {
console.log(error);
});
- Implement a load balancer: A load balancer is a tool that distributes incoming requests across multiple nodes in the distributed system. We can use a tool like NGINX, an open-source web server, to perform load balancing and ensure that each node receives an equal share of the traffic.
We will see an example of how to use the build it cluster module to simulate a load balancer:
const cluster = require('cluster');
const http = require('http');
const numCPUs = require('os').cpus().length;
if (cluster.isMaster) {
console.log(`Master ${process.pid} is running`);
// Fork workers for each available CPU core
for (let i = 0; i < numCPUs; i++) {
cluster.fork();
}
// Listen for worker exit events and restart them
cluster.on('exit', (worker, code, signal) => {
console.log(`worker ${worker.process.pid} died`);
cluster.fork();
});
} else {
// Each worker listens on a different port
http.createServer((req, res) => {
res.writeHead(200);
res.end(`Hello from worker ${process.pid}`);
}).listen(3000 + cluster.worker.id - 1);
console.log(`Worker ${process.pid} started`);
}
When a client makes a request to the application, the request is received by the master process and load balanced to one of the worker processes. Each worker process handles a portion of the incoming requests, thereby distributing the load across all available CPU cores.
If a worker process crashes or exits for any reason, the master process detects this and restarts the worker process, ensuring that the application remains available and responsive.
- Use a database cluster: A database cluster is a group of databases that work together to store and manage data. By using a database cluster, you can ensure that your data is always available and that there are multiple copies of your data in case of failure. MongoDB is a popular NoSQL database that provides clustering and replication features.
Conclusion
Distributed systems are complex but necessary for building highly scalable and fault-tolerant applications. By following the principles of decentralization, redundancy, and consistency and using tools like message queues, load balancers, and database clusters, you can build a distributed system that can handle millions of requests while ensuring that the application is highly available and fault-tolerant. With Node.js, building distributed systems has become easier, and you can leverage its strengths to build powerful and efficient distributed systems.