The power of a trie
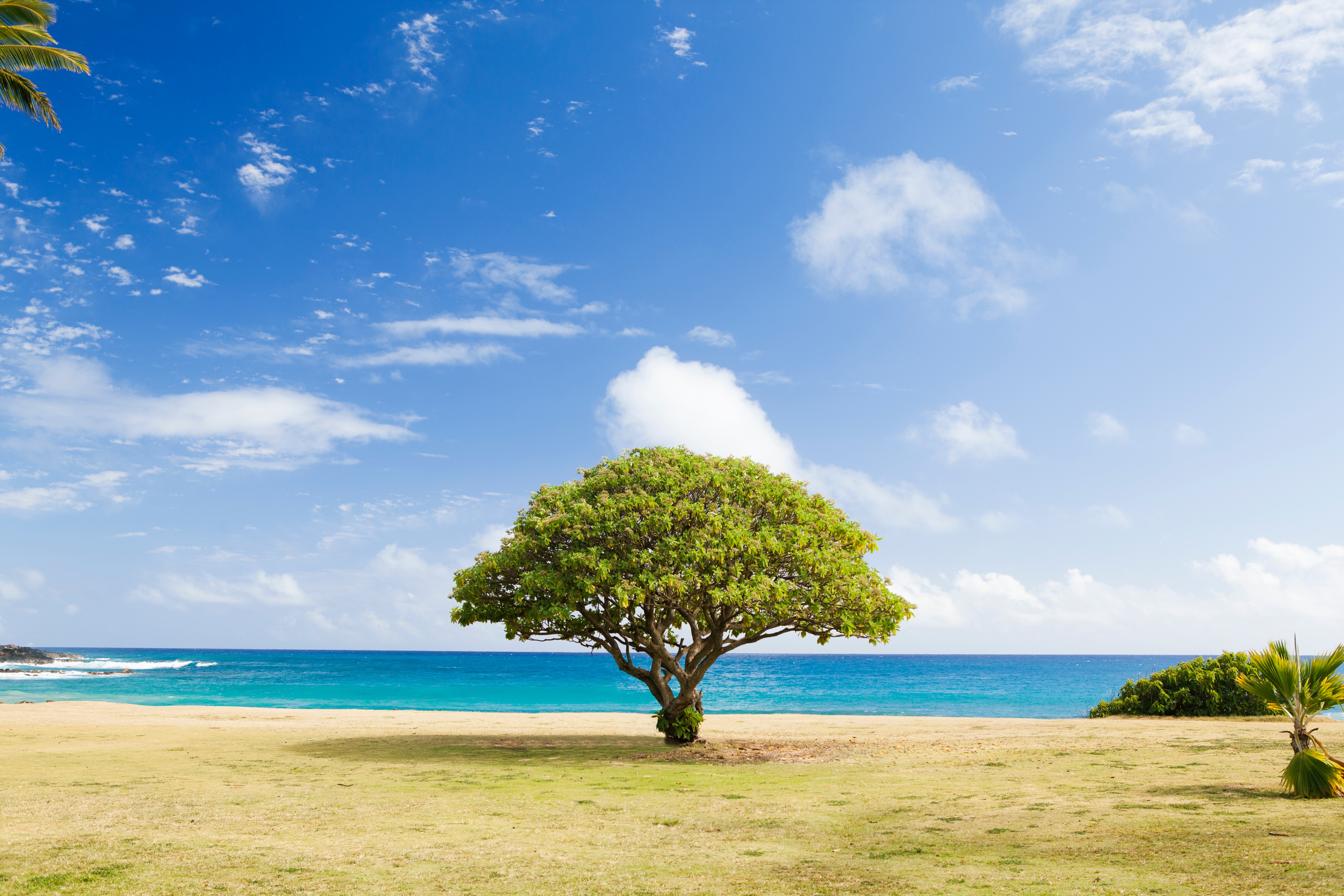
Trie data structure, also known as Prefix Tree, is an efficient data structure used for storing and retrieving a collection of strings. It can be used for tasks such as searching, auto-completion, and spell-checking, etc. The word “trie” is derived from “retrieval,” which is the main purpose of this data structure.
The Trie data structure is based on the tree structure, where each node of the tree represents a single character of a string. The path from the root to the node represents the prefix of the string. Each node can have multiple children, one for each possible character in the alphabet. The leaf nodes of the tree represent complete strings.
Applications of Trie Data Structure:
Trie data structure is used in various applications such as:
-
Spell-Checking: It is used in spell-checking applications to suggest correct spellings for misspelled words.
-
Auto-Completion: It is used in text editors and search engines to suggest the next possible words in real-time based on the entered characters.
-
Routing Tables: It is used in network routing tables to find the next hop for a given IP address.
-
Data Compression: It is used in data compression algorithms like Huffman coding and Lempel-Ziv-Welch algorithm.
Efficient String Searching:
The Trie data structure is very efficient for searching for strings because it only takes O(m) time to find a string of length m. The search algorithm starts from the root of the Trie and follows the path based on the characters of the string until it reaches the leaf node. If the leaf node represents a complete string, then the search is successful. Otherwise, the string is not in the Trie.
class TrieNode {
constructor() {
this.children = new Map();
this.isEndOfWord = false;
}
}
class Trie {
constructor() {
this.root = new TrieNode();
}
insert(word) {
let node = this.root;
for (let char of word) {
if (!node.children.has(char)) {
node.children.set(char, new TrieNode());
}
node = node.children.get(char);
}
node.isEndOfWord = true;
}
search(word) {
let node = this.root;
for (let char of word) {
if (!node.children.has(char)) {
return false;
}
node = node.children.get(char);
}
return node.isEndOfWord;
}
startsWith(prefix) {
let node = this.root;
for (let char of prefix) {
if (!node.children.has(char)) {
return false;
}
node = node.children.get(char);
}
return true;
}
autoCompletion(prefix) {
let node = this.root;
let words = [];
for (let char of prefix) {
if (!node.children.has(char)) {
return words;
}
node = node.children.get(char);
}
this.getWords(node, prefix, words);
return words;
}
getWords(node, prefix, words) {
if (node.isEndOfWord) {
words.push(prefix);
}
for (let [char, child] of node.children) {
this.getWords(child, prefix + char, words);
}
}
}
// Example usage
const trie = new Trie();
trie.insert("apple");
trie.insert("banana");
trie.insert("orange");
trie.insert("pineapple");
console.log(trie.search("apple")); // true
console.log(trie.search("kiwi")); // false
console.log(trie.startsWith("ba")); // true
console.log(trie.startsWith("ki")); // false
console.log(trie.autoCompletion("pi")); // ["pineapple"]
In this implementation, the Trie class has four methods:
- insert(word): Inserts a word into the Trie data structure.
- search(word): Searches for a word in the Trie data structure and returns true if the word is found, false otherwise.
- startsWith(prefix): Checks if any word in the Trie data structure starts with the given prefix and returns true if at least one word is found, false otherwise.
- autoCompletion(prefix): Returns an array of all the words in the Trie data structure that start with the given prefix.
The TrieNode class represents a node in the Trie data structure, which has a children property that is a Map containing the children nodes, and an isEndOfWord property that is true if the node represents the end of a word, and false otherwise.
To insert a word into the Trie data structure, we start from the root node and iterate through each character in the word. For each character, we check if there is a child node with that character, and if not, we create a new child node. We then move to the child node and repeat the process for the next character. Once we have processed all the characters in the word, we set the isEndOfWord property of the final node to true.
To search for a word in the Trie data structure, we start from the root node and iterate through each character in
Representing millions of strings:
The Trie data structure is very efficient for representing millions of strings because it uses a tree structure to store the strings. The Trie can be built in O(n) time, where n is the total number of characters in all the strings. Therefore, the Trie is very efficient for representing a large number of strings.
Comparison with k-ary Search Tree:
The k-ary search tree is another data structure that can be used to represent a collection of strings. The k-ary search tree is similar to the binary search tree, except that each node has k children instead of two. The value of k can be any positive integer.
The k-ary search tree is efficient for searching for strings because it can use binary search to search for the strings. However, the k-ary search tree is not efficient for representing a large number of strings because it uses a linear structure to store the strings. Therefore, the k-ary search tree is not efficient for representing a large number of strings.
Relationship between k-ary Search Tree and Trie:
The Trie data structure and the k-ary search tree are related because they both use a tree structure to represent the strings. However, the Trie data structure is more efficient for representing a large number of strings because it uses a tree structure to store the strings. The k-ary search tree is efficient for searching for strings because it can use binary search to search for the strings.
Conclusion
In conclusion, the Trie data structure is an efficient data structure used for storing and retrieving a collection of strings. It is very efficient for searching for strings because it only takes O(m) time to find a string of length m. The Trie is also very efficient for representing a large number of strings because it uses a tree structure to store the strings. On the other hand, the k-